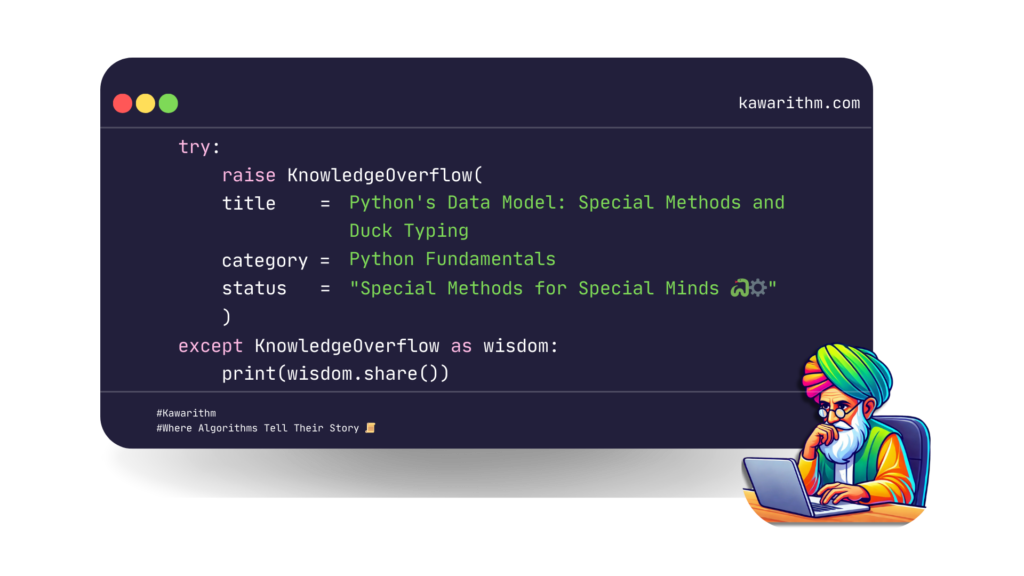
A Deep Dive into Python’s Magic Methods and Pythonic Design
In the heart of Python lies a powerful yet elegant feature known as the Data Model – a framework that allows developers to tap into the language’s internal operations. Through special methods (also known as magic or dunder methods), we can create objects that seamlessly integrate with Python’s built-in functions and operators. Combined with duck typing, this creates a flexible and expressive programming paradigm that’s uniquely Pythonic.
Understanding Special Methods
Special methods in Python are surrounded by double underscores (e.g., __init__
, __str__
), earning them the nickname “dunder methods.” These methods allow objects to implement standard Python operations and integrate with built-in functions.
Basic Object Representation
Let’s start with a simple example that demonstrates the most common special methods:
class Book:
def __init__(self, title, author, pages):
self.title = title
self.author = author
self.pages = pages
def __str__(self):
return f"{self.title} by {self.author}"
def __repr__(self):
return f'Book(title="{self.title}", author="{self.author}", pages={self.pages})'
def __len__(self):
return self.pages
# Usage example
book = Book("The Python Way", "Guido van Rossum", 342)
print(str(book)) # The Python Way by Guido van Rossum
print(repr(book)) # Book(title="The Python Way", author="Guido van Rossum", pages=342)
print(len(book)) # 342
Implementing Container Behavior
class Library:
def __init__(self):
self.books = {}
def __getitem__(self, isbn):
return self.books[isbn]
def __setitem__(self, isbn, book):
if not isinstance(book, Book):
raise TypeError("Can only add books to library")
self.books[isbn] = book
def __contains__(self, isbn):
return isbn in self.books
def __iter__(self):
return iter(self.books.values())
# Usage example
library = Library()
book = Book("Python Cookbook", "David Beazley", 706)
library["978-1449340377"] = book
# Now we can use natural Python syntax
print("978-1449340377" in library) # True
for book in library:
print(book) # Python Cookbook by David Beazley
Operator Overloading and Rich Comparison
Python allows objects to define their behavior for standard operators through special methods:
class Temperature:
def __init__(self, celsius):
self.celsius = celsius
def __add__(self, other):
if isinstance(other, (int, float)):
return Temperature(self.celsius + other)
if isinstance(other, Temperature):
return Temperature(self.celsius + other.celsius)
return NotImplemented
def __eq__(self, other):
if not isinstance(other, Temperature):
return NotImplemented
return self.celsius == other.celsius
def __lt__(self, other):
if not isinstance(other, Temperature):
return NotImplemented
return self.celsius < other.celsius
def __str__(self):
return f"{self.celsius}°C"
# Usage example
t1 = Temperature(25)
t2 = Temperature(30)
print(t1 + 5) # 30°C
print(t1 + t2) # 55°C
print(t1 < t2) # True
Duck Typing in Action
Duck typing is a programming concept where the type or class of an object is less important than the methods it defines. “If it walks like a duck and quacks like a duck, then it must be a duck.”
Here’s a practical example showing how duck typing enables flexible design:
class CSVSerializer:
def serialize(self, data):
if hasattr(data, '__iter__') and hasattr(data, '__len__'):
# We don't care about the specific type, only that it's iterable and has length
return f"Total items: {len(data)}\n" + "\n".join(str(item) for item in data)
raise TypeError("Data must be iterable and have length")
# This works with any iterable that has length
print(CSVSerializer().serialize([1, 2, 3]))
print(CSVSerializer().serialize((4, 5, 6)))
print(CSVSerializer().serialize({7, 8, 9}))
Context Managers with __enter__
and __exit__
Context managers are a powerful feature enabled by special methods:
class DatabaseConnection:
def __init__(self, host):
self.host = host
self.connected = False
def __enter__(self):
print(f"Connecting to {self.host}...")
self.connected = True
return self
def __exit__(self, exc_type, exc_val, exc_tb):
print("Closing connection...")
self.connected = False
# Return True to suppress exceptions, False to propagate them
return False
def query(self, sql):
if not self.connected:
raise RuntimeError("Not connected!")
return f"Executing: {sql}"
# Usage example
with DatabaseConnection("localhost:5432") as db:
result = db.query("SELECT * FROM users")
print(result)
Best Practices and Tips
- Be Consistent: If you implement
__eq__
, consider implementing__hash__
for dictionary keys. - Return NotImplemented: When operator overloading methods can’t handle an operation.
- Document Special Methods: They’re part of your class’s public interface.
- Follow the Principle of Least Surprise: Make special methods behave intuitively.
Conclusion
Python’s data model and duck typing create a powerful foundation for writing clean, intuitive code. By understanding and properly implementing special methods, you can create classes that feel like they’re part of Python itself. Remember that with great power comes great responsibility – use these features judiciously to enhance your code’s readability and maintainability.
Duck typing, combined with special methods, enables a style of programming that’s both flexible and expressive. Instead of rigid hierarchies, we can focus on behavior and capabilities, leading to more adaptable and maintainable code.
This deep integration with Python’s core features is what makes the language special, allowing developers to write code that’s both powerful and beautiful.