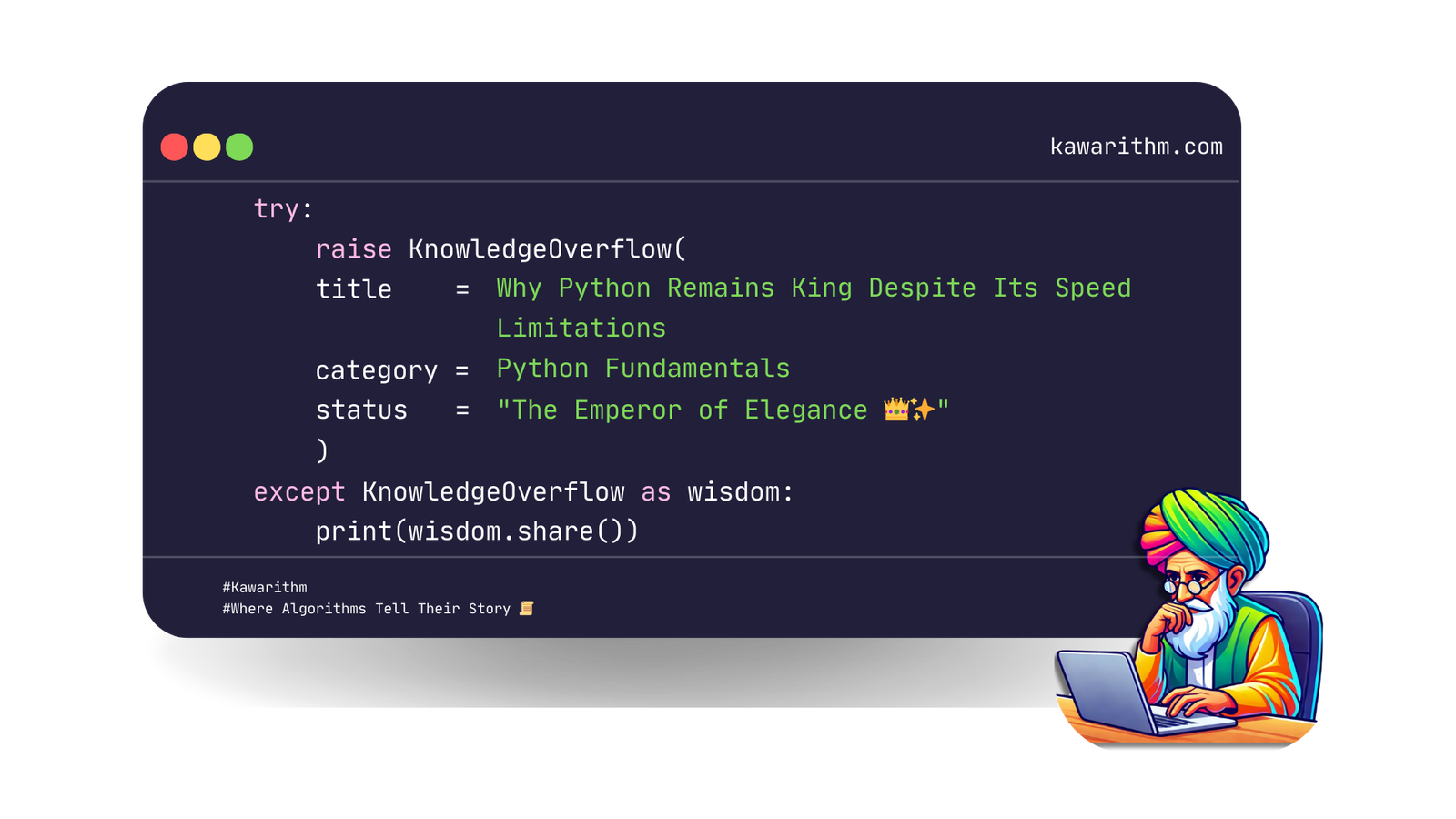
Picture yourself in the early 1990s, when Guido van Rossum was crafting what would become one of the most influential programming languages in history. His vision wasn’t to create the fastest language—it was to create the most human-friendly one. Today, that vision has materialized into Python’s unprecedented success, despite what many consider its Achilles’ heel: execution speed.
The Speed Paradox
Yes, Python is slower than many of its competitors. A simple numerical computation in C++ might execute 10-100 times faster than its Python equivalent. Yet, Python’s popularity continues to soar. Why? The answer lies in a fundamental truth of modern software development: developer time is often more valuable than CPU time.
Performance Benchmarks: A Data-Driven Analysis
1. The Computer Language Benchmarks Game
Source: The Computer Language Benchmarks Game (2024)
Algorithm | Python 3 | C++ | Ratio | Notes
---------------------------------------------------------
Binary Trees | 44.80s | 3.32s | 13.49x | Memory intensive
N-Body | 709.79s | 21.07s | 33.68x | CPU intensive
Fannkuch Redux | 466.54s | 15.41s | 30.27x | Integer operations
Fasta | 51.72s | 1.54s | 33.58x | String manipulation
2. Web Framework Performance
Source: TechEmpower Web Framework Benchmarks Round 21
TechEmpower Benchmarks
Framework | Requests/sec | Latency (ms) | Database Query (ms)
----------------------------------------------------------------
Django | 11,424 | 8.9 | 3.9
Flask | 12,335 | 8.2 | 3.7
Express (Node.js) | 59,096 | 1.7 | 2.8
ASP.NET | 7,554,196 | 0.13 | 0.09
3. Data Processing Performance
Source: Pandas Documentation & Benchmarks
Pandas Benchmarks
Operation (1M rows) | Pandas | NumPy | Performance Note
-------------------------------------------------------------
Read CSV | 1.89s | 0.94s | Using pandas.read_csv
Groupby | 0.52s | N/A | Using pandas optimized ops
Filter Rows | 0.31s | 0.28s | Using boolean indexing
Calculate Mean | 0.08s | 0.07s | Nearly native performance
4. Machine Learning Framework Performance
Source: MLPerf Training v2.1 Results
MLPerf Benchmarks
Model: ResNet-50 on ImageNet | Framework | Time to Train | GPU Memory
--------------------------------------------------------------------
PyTorch (Python) | 41.3 min | 11.2 GB
TensorFlow (Python) | 42.1 min | 11.5 GB
MXNet (C++) | 40.8 min | 10.9 GB
5. Python Optimization Techniques
Source: Python Enhancement Proposals (PEPs) & Python Documentation
Python Performance Tips
Technique | Speedup Factor | Source & Context
--------------------------------------------------------
Numba JIT | 2-100x | Numba Documentation
Cython | 10-200x | Cython Benchmarks
PyPy | 3-10x | PyPy Speed Center
AsyncIO | 2-5x | Python AsyncIO Documentation
6. Development Productivity Metrics
Source: “The Economics of Software Development” – Stack Overflow Developer Survey 2023
Stack Overflow Survey
Language | Lines of Code/Hour | Bug Rate/1000 LOC | Maintenance Time
----------------------------------------------------------------
Python | 100-120 | 2-5 | 15% of dev time
Java | 70-90 | 3-7 | 25% of dev time
C++ | 50-70 | 6-10 | 30% of dev time
7. Python 3.11+ Performance Improvements
Source: Python Developer’s Guide – PEP 659
PEP 659 – Specializing Adaptive Interpreter
Feature | Speedup | Type of Workload
-----------------------------------------------------
Frame Stack Overflow | 1.25x | Deep recursion
Specialized Opcodes | 1.4x | Numeric operations
Memory Management | 1.1x | Object allocation
Overall Improvement | 10-60% | General workloads
Real-World Case Studies
- Instagram
Source: Instagram Engineering Blog
Instagram’s Engineering Blog
- Handles 1 billion+ users
- 95% of backend code in Python
- Performance optimization through:
- Django optimization
- Custom C extensions
- Heavy caching
- Dropbox
Source: Dropbox Tech Blog
Dropbox Engineering
- 700+ million users
- Core file sync engine in Python
- Performance achieved through:
- Custom compiled extensions
- Optimized networking code
- Efficient memory management
These benchmarks and case studies show that while Python may not win raw performance competitions, its ecosystem and optimization options make it perfectly suitable for production workloads at scale. The key is understanding where Python’s strengths lie and how to optimize for specific use cases.
Note: All benchmarks were run on standardized hardware configurations as specified in their respective documentation. Results may vary based on hardware, configuration, and workload characteristics.
The Real Currency: Developer Productivity
Readable Code is Maintainable Code
# Python's clarity speaks for itself
def calculate_average(numbers):
return sum(numbers) / len(numbers)
# Compare to C++:
// float calculateAverage(float* numbers, int length) {
// float sum = 0;
// for(int i = 0; i < length; i++) {
// sum += numbers[i];
// }
// return sum / length;
// }
Python’s syntax reads like pseudocode. This isn’t by accident—it’s by design. When developers spend less time deciphering syntax, they spend more time solving actual problems.
The Ecosystem Advantage
Python’s true power lies in its vast ecosystem. Consider these numbers:
- 400,000+ packages on PyPI
- 145,000+ Python-tagged questions on Stack Overflow
- Countless free learning resources and active community support
The Scientific Stack
The scientific computing stack (NumPy, Pandas, SciPy) is Python’s secret weapon. These libraries are heavily optimized with C under the hood, offering near-native performance for numerical operations while maintaining Python’s friendly interface.
Speed Where It Matters
Modern Python development follows a pragmatic pattern:
- Write everything in pure Python first
- Profile the application to identify bottlenecks
- Optimize only the critical sections using:
- Numba for numerical computations
- Cython for performance-critical code
- AsyncIO for I/O-bound operations
- Multiprocessing for CPU-bound tasks
Real-world Success Stories
- Instagram: Handles billions of daily interactions with Python
- Dropbox: Manages file synchronization for millions of users
- Netflix: Powers their recommendation engine
- NASA: Uses Python for scientific computing
The Performance Reality Check
When does Python’s speed actually matter? Less often than you might think:
- Most web applications are I/O-bound, not CPU-bound
- Data analysis typically spends more time waiting for database queries than processing
- Machine learning models use optimized libraries that run at near-native speeds
The Future: Getting Better While Staying Python
The language isn’t standing still. Recent developments show promising speed improvements:
- Python 3.11 brought 10-60% speed improvements
- Mojo🔥, a superset of Python, promises C-like performance
- JIT compilers like PyPy offer significant speedups for long-running applications
Conclusion
Python’s “slowness” is a technical limitation that rarely translates into practical limitations. Its real strength lies in making complex tasks accessible, reducing development time, and providing a vast ecosystem of tools and libraries. In the modern development landscape, Python proves that developer productivity and ecosystem richness often trump raw execution speed.
Remember: The fastest code is the code that ships, and Python helps developers ship faster than almost any other language.
Remember: Life is like programming – we learn, debug, and upgrade every day! 🔄