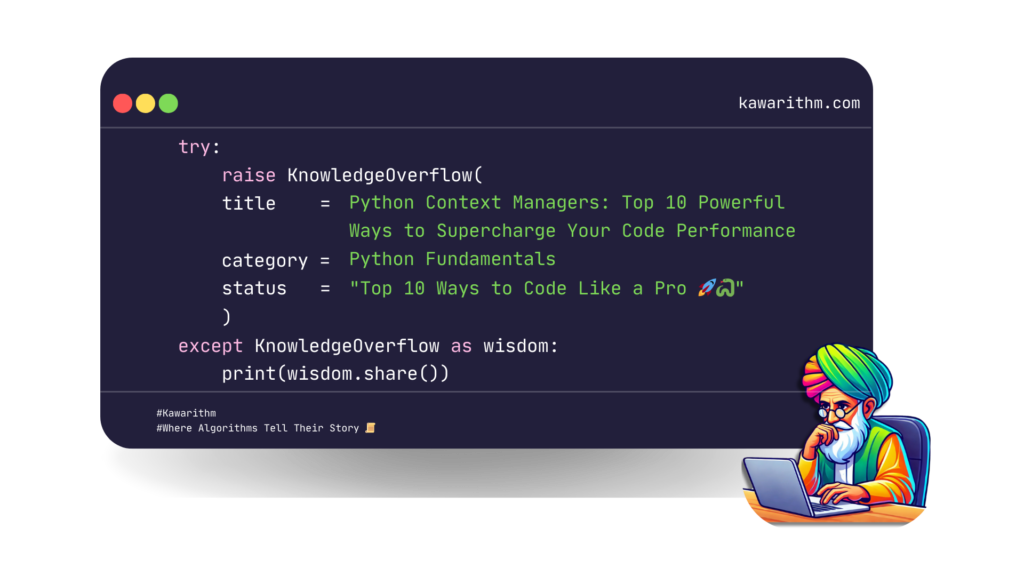
Introduction: The Magic Behind Python Context Managers
Python context managers are one of those powerful features that separate intermediate developers from advanced ones. They’re not just about the famous with
statement for file handling – they’re your secret weapon for writing cleaner, faster, and more maintainable code. Let’s dive into the top 10 ways you can leverage context managers to boost your Python programming.
1. The Classic File Handler: Beyond the Basics
Understanding the Foundation
# Traditional approach - prone to resource leaks
file = open('data.txt', 'r')
try:
content = file.read()
finally:
file.close()
# Context manager approach - clean and safe
with open('data.txt', 'r') as file:
content = file.read()
# File automatically closes, even if exceptions occur
Why It’s Powerful
- Automatic resource cleanup
- Exception-safe handling
- Reduced boilerplate code
- Memory efficiency through immediate release
2. Database Connections: Ensuring Clean Transactions
Managing SQL Connections
from contextlib import contextmanager
import sqlite3
@contextmanager
def database_connection():
conn = sqlite3.connect('example.db')
try:
yield conn
conn.commit()
except Exception:
conn.rollback()
raise
finally:
conn.close()
# Usage
with database_connection() as conn:
cursor = conn.cursor()
cursor.execute("SELECT * FROM users")
3. Threading Locks: Thread-Safe Operations
Concurrent Programming Made Easy
from threading import Lock
class ThreadSafeCounter:
def __init__(self):
self._counter = 0
self._lock = Lock()
@contextmanager
def atomic_increment(self):
with self._lock:
try:
yield self._counter
self._counter += 1
finally:
pass # Lock is automatically released
counter = ThreadSafeCounter()
with counter.atomic_increment() as value:
print(f"Current value: {value}")
4. Timer Context: Performance Monitoring
Measuring Code Execution Time
from time import perf_counter
from contextlib import contextmanager
@contextmanager
def timer(description: str):
start = perf_counter()
yield
elapsed = perf_counter() - start
print(f"{description}: {elapsed:.3f} seconds")
# Usage
with timer("Matrix multiplication"):
# Your expensive computation here
result = calculate_matrix()
5. Memory Management: Temporary Resource Allocation
Efficient Resource Handling
class TempDirectory:
def __init__(self, path):
self.path = path
def __enter__(self):
import os
os.makedirs(self.path, exist_ok=True)
return self.path
def __exit__(self, exc_type, exc_val, exc_tb):
import shutil
shutil.rmtree(self.path)
# Usage
with TempDirectory("/tmp/workdir") as temp_path:
# Work with temporary files
pass # Directory is automatically cleaned up
6. Network Connections: Socket Management
Clean Network Programming
import socket
@contextmanager
def tcp_connection(host, port):
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
try:
sock.connect((host, port))
yield sock
finally:
sock.close()
# Usage
with tcp_connection("example.com", 80) as conn:
conn.send(b"GET / HTTP/1.1\r\n\r\n")
response = conn.recv(1024)
7. Redirecting Output: Testing and Debugging
Capturing Standard Output
from io import StringIO
import sys
from contextlib import contextmanager
@contextmanager
def captured_output():
new_out = StringIO()
old_out = sys.stdout
try:
sys.stdout = new_out
yield new_out
finally:
sys.stdout = old_out
# Usage
with captured_output() as output:
print("Hello, World!")
captured = output.getvalue()
8. Environment Variables: Temporary Settings
Managing Environment State
import os
from contextlib import contextmanager
@contextmanager
def environment_variable(key, value):
original = os.environ.get(key)
os.environ[key] = value
try:
yield
finally:
if original is None:
del os.environ[key]
else:
os.environ[key] = original
# Usage
with environment_variable("API_KEY", "test_key"):
# Code that needs specific environment variable
pass
9. Numpy Array Operations: Memory Optimization
Efficient Array Processing
import numpy as np
from contextlib import contextmanager
@contextmanager
def temporary_array_copy(array):
temp = array.copy()
try:
yield temp
finally:
del temp # Explicitly free memory
# Usage
original = np.array([1, 2, 3])
with temporary_array_copy(original) as temp:
temp += 1 # Modify without affecting original
10. Nested Context Managers: Advanced Patterns
Combining Multiple Contexts
from contextlib import ExitStack
def complex_operation():
with ExitStack() as stack:
files = [
stack.enter_context(open(f'file{i}.txt'))
for i in range(3)
]
# Work with multiple files simultaneously
return [f.read() for f in files]
Best Practices and Performance Tips
When to Use Context Managers
- Resource management (files, connections, locks)
- Setup and teardown operations
- State changes that need to be reverted
- Performance monitoring and profiling
Performance Considerations
- Use context managers for deterministic cleanup
- Avoid nested context managers unless necessary
- Implement
__enter__
and__exit__
methods efficiently - Consider using
contextlib.contextmanager
for simple cases
Additional Resources
Official Documentation and References
- Python Context Manager Documentation – The official Python documentation covering the context manager protocol, including detailed explanations of
__enter__
and__exit__
methods. - contextlib — Utilities for with-statement contexts – Comprehensive guide to the
contextlib
module, which provides utilities for common tasks involving thewith
statement. - PEP 343 — The “with” Statement – The original Python Enhancement Proposal that introduced the
with
statement, providing deep insights into its design and rationale.
Conclusion
Python context managers are more than just syntactic sugar – they’re a powerful tool for writing robust, maintainable code. By implementing these patterns in your projects, you’ll not only improve code reliability but also boost performance through proper resource management.