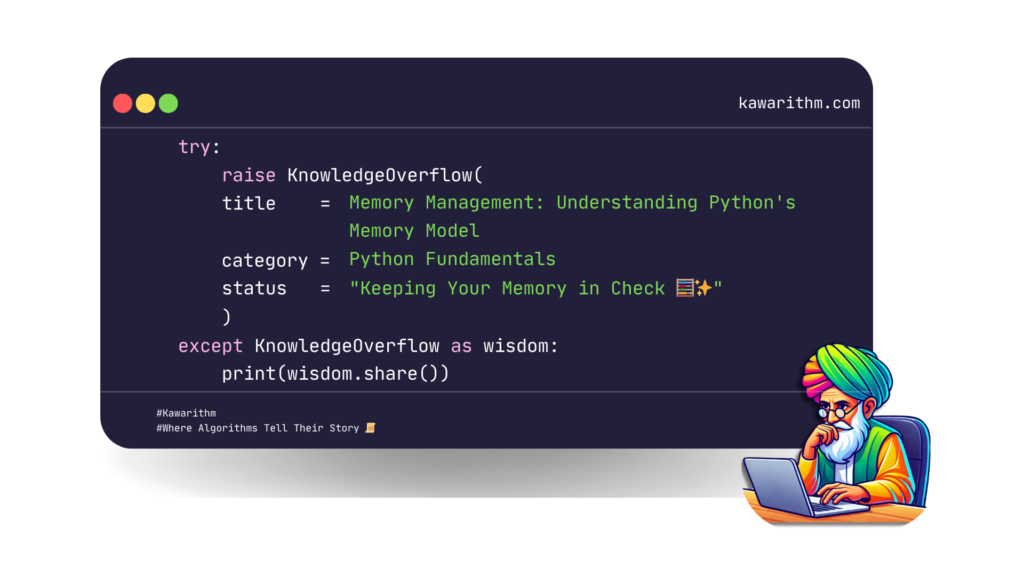
A Friendly Guide for Non-Programmers
Imagine you’re organizing a busy restaurant kitchen. Every chef needs a workspace, ingredients need to be stored properly, and dirty dishes must be cleaned up. This is very similar to how Python manages computer memory! Let’s explore this fascinating world together.
The Kitchen Counter (Memory Space)
Think of your computer’s memory like a giant kitchen counter. When you run a Python program, it’s like starting a busy dinner service. You need space for all your ingredients (data) and cooking preparations (operations).
# Creating variables is like putting ingredients on the counter
name = "Gordon" # A single ingredient (string)
menu_items = [1, 2, 3] # A tray of ingredients (list)
The Chef’s Workspace (Variable Scope)
In a restaurant, different chefs work in different stations. Similarly, Python organizes its code into different “workspaces” called scopes. Let’s see this in action:
def prepare_appetizer():
# This ingredient (variable) only exists in the appetizer station
sauce = "special sauce"
print(f"Preparing appetizer with {sauce}")
# We can't access 'sauce' out here!
# This would cause an error:
# print(sauce) # ❌ Error!
Think of it this way: A prep cook working on desserts can’t grab ingredients from the appetizer station without asking. Each station (scope) keeps its ingredients (variables) organized and separate.
The Cleanup Crew (Garbage Collection)
Here’s where Python really shines! Remember those dirty dishes in the kitchen? In programming, we create lots of “dirty dishes” (unused data) that take up space. Python has an amazing automatic cleanup crew called the Garbage Collector.
Let’s see how it works:
def serve_meal():
# Create a temporary shopping list
shopping_list = ["tomatoes", "lettuce", "onions"]
print("Order taken!")
# Once this function ends, we don't need the shopping list anymore
# Python's cleanup crew (garbage collector) automatically removes
# the shopping_list since it's no longer needed
Just like a good restaurant bus staff clearing tables without being asked, Python’s garbage collector automatically identifies and removes data that’s no longer being used. You don’t have to worry about cleaning up – Python handles it!
Memory Management Tips (Best Practices)
- Clean As You Go
# Instead of:
big_list = list(range(1000000))
# Process the list...
# Let garbage collector clean it
# Better approach:
for number in range(1000000):
# Process one item at a time
process(number)
- Use Context Managers
Just like having a dishwasher that automatically starts and stops:
# Python automatically handles cleanup
with open("recipe.txt", "r") as recipe_file:
contents = recipe_file.read()
# File is automatically closed when we're done
Common Memory Pitfalls (Kitchen Disasters to Avoid)
- Circular References
Imagine two chefs each waiting for the other’s station to be free:
def create_circular_mess():
chef_a = {"name": "Alice"}
chef_b = {"name": "Bob"}
chef_a["partner"] = chef_b # Alice depends on Bob
chef_b["partner"] = chef_a # Bob depends on Alice
- Large Objects in Memory
Like preparing too many dishes at once:
# Be careful with this:
all_orders = [order for order in get_all_orders()] # Loads everything at once
# Better approach:
for order in get_all_orders():
process_single_order(order) # Handle one at a time
Final Thoughts
Python’s memory management is like having a well-organized kitchen with an excellent staff. The chef (you) focuses on creating amazing dishes (writing code), while Python handles the workspace organization (variable scope) and cleanup (garbage collection).
Remember:
- Variables are like ingredients on your counter
- Scopes are like different cooking stations
- The garbage collector is your cleanup crew
- Python handles most of the memory management automatically
Just like a good chef focuses on cooking great food rather than washing dishes, Python lets you focus on writing great code while it handles the memory cleanup behind the scenes!