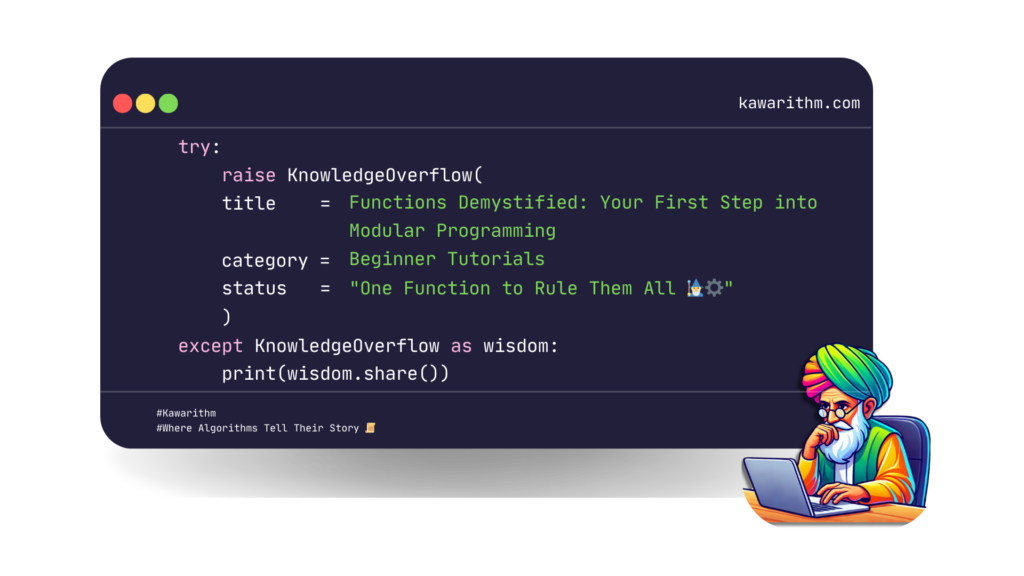
Picture yourself as a master chef in a bustling kitchen. Each day, you prepare the same exquisite chocolate soufflé for your guests. Instead of explaining the recipe step-by-step to every new kitchen apprentice, you’ve written it down once, perfected it, and now anyone can follow it to create the same delightful dessert. This is exactly what functions do in programming – they’re your tried-and-true recipes that can be used whenever needed.
The Birth of a Function
Let’s start with a simple story. Sarah, a budding programmer, found herself writing the same code over and over to greet her program’s users. Each time, she wrote:
user_name = input("What's your name? ")
print(f"Hello, {user_name}! Welcome to our adventure!")
print("We're excited to have you here!")
After the fifth time, she thought, “There must be a better way!” And thus, her first function was born:
def greet_adventurer():
user_name = input("What's your name? ")
print(f"Hello, {user_name}! Welcome to our adventure!")
print("We're excited to have you here!")
Just like that, Sarah had created her first reusable piece of code. The def
keyword was like drawing a box around her recipe, giving it a name, and putting it on the shelf for later use. Whenever she needed to greet someone, she could simply call greet_adventurer()
, and her function would spring into action.
Adding Ingredients: Function Arguments
As Sarah’s program grew, she realized she wanted to greet people differently based on their role in the adventure. This is where function arguments came into play – think of them as ingredients you can vary in your recipe:
def greet_adventurer(name, role):
print(f"Welcome, {name} the {role}!")
if role == "Wizard":
print("May your spells be powerful!")
elif role == "Warrior":
print("May your sword strike true!")
else:
print("May fortune favor your journey!")
Now Sarah could write:
greet_adventurer("Gandalf", "Wizard")
greet_adventurer("Aragorn", "Warrior")
Each call to the function produced a unique greeting, tailored to the character. The arguments name
and role
were like slots in her recipe that could be filled with different values each time.
Returning the Treasure: Return Values
But what if Sarah wanted her function to do more than just print messages? What if she wanted it to create something she could use later? Enter return values – the treasures that functions can give back:
def create_character(name, role):
character = {
"name": name,
"role": role,
"level": 1,
"skills": []
}
if role == "Wizard":
character["skills"] = ["Fireball", "Shield"]
elif role == "Warrior":
character["skills"] = ["Slash", "Block"]
return character
# Creating a new character
gandalf = create_character("Gandalf", "Wizard")
print(f"{gandalf['name']} knows these skills: {gandalf['skills']}")
The return
statement is like packaging up the result of your recipe to be used elsewhere. In this case, create_character()
returns a dictionary containing all the information about the new character.
The Magic of Default Arguments
As Sarah’s adventure game grew more complex, she realized some arguments should have default values – like a recipe that suggests certain ingredients but allows substitutions:
def create_character(name, role="Adventurer", level=1):
character = {
"name": name,
"role": role,
"level": level,
"skills": ["Basic Attack"]
}
return character
# These all work!
hero1 = create_character("Frodo") # Uses defaults
hero2 = create_character("Gandalf", "Wizard", 5) # Specifies everything
The Power of Modularity
By the end of her journey, Sarah had discovered the true power of functions: they allowed her to break down complex problems into manageable pieces. Each function was like a well-tested recipe in her cookbook, ready to be used whenever needed.
Remember, just as a master chef builds their reputation on reliable recipes, a programmer builds robust applications on well-designed functions. They’re not just blocks of code – they’re the building blocks of your programming journey, waiting to be combined in endless ways to create something amazing.
The next time you find yourself copying and pasting code, remember Sarah’s story. Ask yourself: “Could this be a function?” Because in the world of programming, functions aren’t just about avoiding repetition – they’re about creating clarity, reliability, and the foundation for greater things to come.