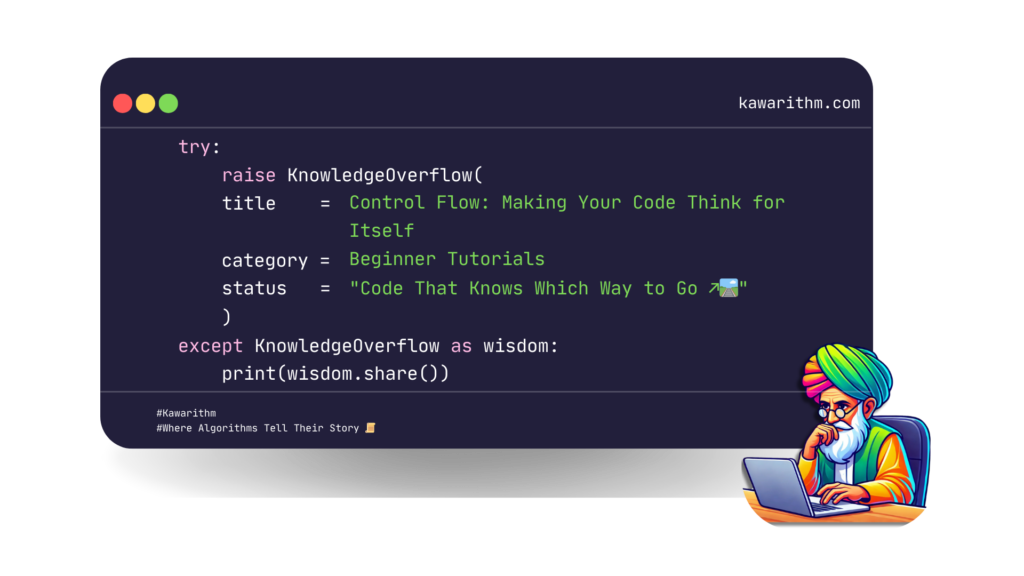
Picture this: You’re standing at the helm of a vast digital ship, navigating through seas of data and logic. Just as a captain needs to make split-second decisions based on weather conditions, your code needs to think and adapt. Welcome to the fascinating world of control flow – where code comes alive with decision-making powers.
Let’s embark on this journey together, starting with the humble if statement, the basic building block of programmatic decision-making.
The Guardian at the Gate: If Statements
Imagine you’re a bouncer at an exclusive club called “Code Execution.” Your job? Making sure only the right conditions get through. Here’s how it looks in Python:
age = 17
if age >= 18:
print("Welcome to the club!")
else:
print("Come back in a few years!")
But life (and code) isn’t always black and white. Sometimes we need multiple conditions, like a sophisticated security system. Enter the elif statement:
score = 85
if score >= 90:
grade = "A"
elif score >= 80:
grade = "B"
elif score >= 70:
grade = "C"
else:
grade = "Need improvement"
The Time Loop: While and For Loops
Now, picture yourself in a time loop, like Groundhog Day. That’s essentially what while loops are – repeating actions until a condition is met:
attempts = 0
while attempts < 3:
password = input("Enter password: ")
if password == "secret":
print("Access granted!")
break
attempts += 1
But what about for loops? They’re like a tour guide, leading you through a predetermined collection of items:
fibonacci = [1, 1, 2, 3, 5, 8, 13]
running_sum = 0
for number in fibonacci:
running_sum += number
print(f"Current sum: {running_sum}")
The Art of Nested Control
Here’s where it gets interesting – like a chess master thinking several moves ahead, we can nest our control structures:
for floor in range(1, 5):
if floor == 13: # Superstitious building
continue
for room in range(1, 5):
if floor == 3 and room == 2: # Maintenance
print(f"Room {floor}0{room}: Under renovation")
continue
print(f"Room {floor}0{room}: Available")
The Secret Weapon: List Comprehensions
And now, let me share a secret weapon in Python’s arsenal. List comprehensions are like control flow poetry:
# Instead of:
squares = []
for i in range(10):
if i % 2 == 0:
squares.append(i ** 2)
# You can write:
squares = [i ** 2 for i in range(10) if i % 2 == 0]
The Guard Clauses: Early Returns
Think of guard clauses as your code’s early warning system. They help you fail fast and maintain clean code:
def process_user_data(user_dict):
if not user_dict:
return "Error: Empty user data"
if 'name' not in user_dict:
return "Error: Name required"
if len(user_dict['name']) < 2:
return "Error: Name too short"
# Process valid user data
return f"Welcome, {user_dict['name']}!"
The Match Statement: The New Kid on the Block
Python 3.10 introduced pattern matching, like a sophisticated switch statement with superpowers:
command = "help"
match command:
case "start":
print("Starting the program")
case "stop":
print("Stopping the program")
case "help":
print("Showing help menu")
case _:
print("Unknown command")
Control flow is more than just syntax – it’s about teaching your code to think. Each if statement is a decision point, each loop a journey, and each condition a guardian of logic. Master these concepts, and you’ll transform from a code writer into a code composer, orchestrating complex symphonies of logic and computation.
Remember, just as a story needs its plot twists and turns to be engaging, your code needs its control structures to be powerful and meaningful. The next time you write an if statement or craft a loop, remember: you’re not just writing code – you’re creating a narrative of logic that will guide your program through its journey.
The beauty of control flow lies not just in making your code work, but in making it think. And that’s where the magic happens.