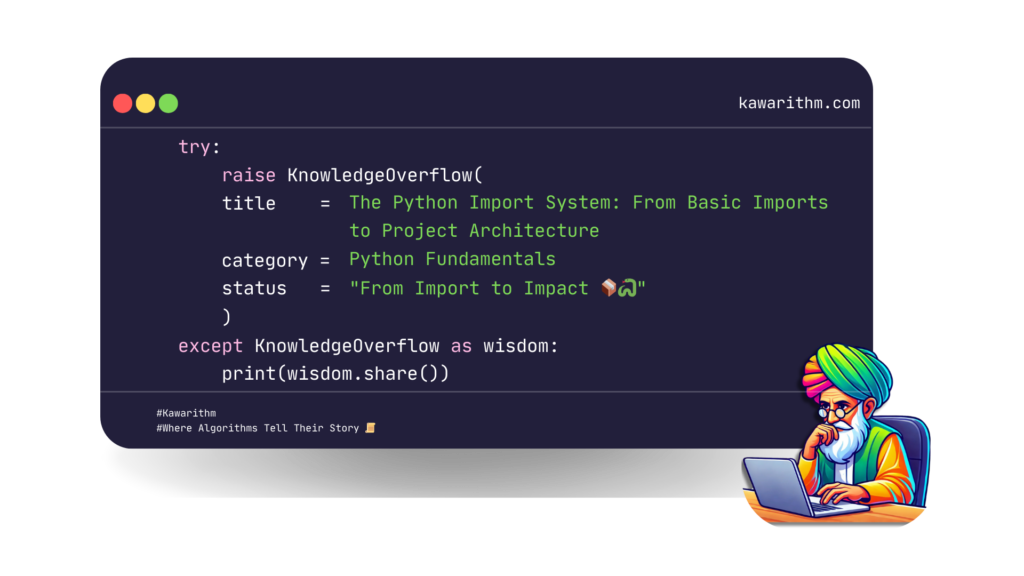
The Journey Begins: Understanding Modules
Imagine Python modules as chapters in a grand book of code. Each .py
file you create is a self-contained story, ready to share its contents with others. Let’s start with a simple tale:
# weather.py
def get_temperature(city):
return f"Getting temperature for {city}"
def get_forecast(city, days):
return f"Forecasting {days} days for {city}"
Now, we can import this story in different ways:
# Method 1: Import the entire module
import weather
print(weather.get_temperature("Tokyo"))
# Method 2: Import specific functions
from weather import get_temperature
print(get_temperature("Tokyo"))
# Method 3: Import with an alias
import weather as w
print(w.get_temperature("Tokyo"))
The Plot Thickens: Understanding Package Hierarchies
As our codebase grows, we need to organize our modules into packages. A package is like a book series, containing multiple related modules. Here’s how we might structure a weather application:
weather_app/
│
├── __init__.py
├── core/
│ ├── __init__.py
│ ├── temperature.py
│ └── forecast.py
├── utils/
│ ├── __init__.py
│ └── validation.py
└── api/
├── __init__.py
└── endpoints.py
Let’s bring this structure to life:
# weather_app/core/temperature.py
class TemperatureSensor:
def __init__(self, location):
self.location = location
def get_reading(self):
return f"Temperature reading from {self.location}"
# weather_app/utils/validation.py
def validate_location(location):
return bool(location and isinstance(location, str))
# weather_app/api/endpoints.py
from ..core.temperature import TemperatureSensor
from ..utils.validation import validate_location
def get_temperature_endpoint(location):
if validate_location(location):
sensor = TemperatureSensor(location)
return sensor.get_reading()
return "Invalid location"
The Plot Twist: Relative vs Absolute Imports
In our story, we can reference other modules using either relative or absolute paths:
# Relative imports (from current package)
from .temperature import TemperatureSensor # Single dot
from ..utils.validation import validate_location # Double dot
# Absolute imports (from project root)
from weather_app.core.temperature import TemperatureSensor
from weather_app.utils.validation import validate_location
The Extended Universe: Virtual Environments
Now, let’s talk about keeping our story’s world contained and reproducible. Virtual environments are like parallel universes where each project can have its own set of dependencies:
# Creating a new virtual environment
python -m venv weather_env
# Activating the environment
# On Windows:
weather_env\Scripts\activate
# On Unix or MacOS:
source weather_env/bin/activate
# Installing project dependencies
pip install requests pandas numpy
# Freezing dependencies
pip freeze > requirements.txt
Your requirements.txt
becomes a recipe for recreating your project’s universe:
requests==2.31.0
pandas==2.1.0
numpy==1.24.3
Advanced Chapters: Import Hooks and Dynamic Imports
Sometimes we need to add some magic to our story. Here’s how we can import modules dynamically:
# Dynamic imports based on configuration
def get_database_client(db_type):
if db_type == "postgres":
import psycopg2
return psycopg2.connect
elif db_type == "mysql":
import mysql.connector
return mysql.connector.connect
else:
raise ValueError(f"Unsupported database: {db_type}")
# Lazy loading modules
def analyze_data():
# Only import pandas when we actually need it
import pandas as pd
return pd.DataFrame({'data': [1, 2, 3]})
The Hidden Powers: Import System Hooks
For advanced use cases, we can even customize how Python imports modules:
# Custom importer for encrypted modules
class EncryptedModuleImporter:
def find_spec(self, fullname, path, target=None):
if fullname.startswith('secret_'):
return importlib.util.spec_from_file_location(
fullname,
f"{fullname}.enc",
loader=EncryptedModuleLoader()
)
return None
# Register the custom importer
import sys
sys.meta_path.append(EncryptedModuleImporter())
Best Practices: Writing Clean Import Stories
- Organize Imports by Type
# Standard library imports
import os
import sys
from datetime import datetime
# Third-party imports
import pandas as pd
import numpy as np
# Local application imports
from .core import temperature
from .utils import validation
- Use
__all__
to Control Exports
# temperature.py
__all__ = ['TemperatureSensor', 'get_temperature']
class TemperatureSensor:
pass
def get_temperature():
pass
def _internal_helper(): # Not exported
pass
- Handle Optional Dependencies
try:
import pandas as pd
HAS_PANDAS = True
except ImportError:
HAS_PANDAS = False
def analyze_data(data):
if HAS_PANDAS:
return pd.DataFrame(data).describe()
else:
return "Pandas is required for data analysis"
Project Structure Template
Here’s a template for a well-organized Python project:
project_root/
├── src/
│ └── package_name/
│ ├── __init__.py
│ ├── core/
│ ├── utils/
│ └── api/
├── tests/
│ ├── __init__.py
│ ├── test_core/
│ ├── test_utils/
│ └── test_api/
├── docs/
├── requirements.txt
├── setup.py
└── README.md
Dependency Management with Poetry
Modern Python projects often use Poetry for dependency management:
# pyproject.toml
[tool.poetry]
name = "weather-app"
version = "0.1.0"
description = "A weather monitoring application"
[tool.poetry.dependencies]
python = "^3.8"
requests = "^2.31.0"
pandas = "^2.1.0"
[tool.poetry.dev-dependencies]
pytest = "^7.4.0"
black = "^23.7.0"
Conclusion: Mastering the Art of Python Imports
The Python import system is much more than just a way to access code from other files – it’s a powerful framework that enables you to build scalable, maintainable, and elegant applications. As we’ve explored throughout this guide, understanding imports is crucial for several reasons:
Key Takeaways
- Modularity is Power: The import system allows you to break down complex applications into manageable, reusable components. Like LEGO blocks, well-structured modules can be assembled in different ways to create various applications.
- Organization Matters: A thoughtful project structure, combined with appropriate use of packages and modules, makes your codebase easier to navigate and maintain. Remember: time spent organizing your imports is an investment in your project’s future.
- Virtual Environments are Your Friends: They keep your projects isolated and dependencies clean. Think of them as separate workshops where each project can have its own set of tools without interfering with others.
- Import Flexibility is a Feature: Python’s various import mechanisms (relative, absolute, dynamic) give you the flexibility to structure your code in the way that best serves your project’s needs.
Moving Forward
As you continue your Python journey, consider these practices:
- Start new projects by planning their structure and import strategy
- Regularly audit your imports to prevent unused or circular dependencies
- Keep your virtual environments clean and requirements updated
- Document your project’s import patterns for other developers
Remember that mastering imports is an iterative process. Begin with simple, clear structures and gradually incorporate more advanced patterns as your needs evolve. The goal isn’t to use every feature of the import system, but to use the right features to make your code more maintainable and efficient.
Final Thoughts
The Python import system is a testament to the language’s “batteries included” philosophy. It provides all the tools you need to organize code from the smallest script to the largest application. By understanding and properly utilizing these tools, you’re not just writing code – you’re crafting software that can grow and adapt with your needs.
Happy coding, and may your imports always be clean and your dependencies resolved! 🐍✨