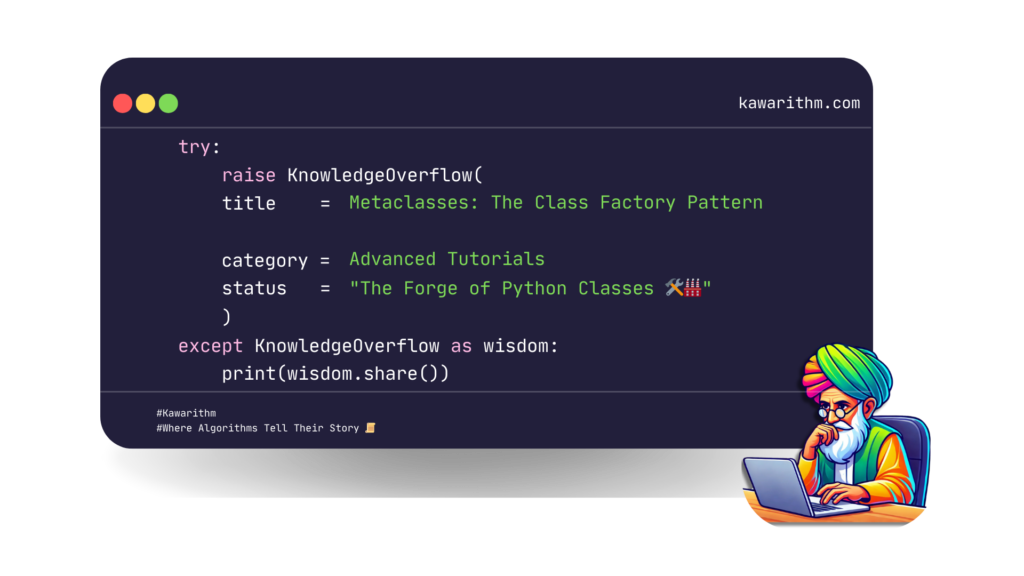
A Deep Dive into Python’s Class Creation Magic
In the grand theater of Python programming, there exists a powerful but often misunderstood feature that operates behind the scenes: metaclasses. Picture them as the master puppeteers of class creation, orchestrating how classes themselves come into being. Today, we’ll pull back the curtain and explore this fascinating world of “classes that create classes.”
The Genesis: Understanding Class Creation
Before we delve into metaclasses, let’s understand a fundamental truth: in Python, everything is an object – even classes themselves. When you write a class definition, Python doesn’t simply stamp it into existence. Instead, it performs an elaborate dance of creation, following a carefully choreographed sequence of steps.
# This seemingly simple class definition...
class MyClass:
pass
# ...is actually more like this behind the scenes
MyClass = type('MyClass', (), {})
Enter the Metaclass: The Master Builder
A metaclass is the architect that designs how your classes are built. It’s the template for creating class objects, just as a class is a template for creating instance objects. Let’s create our first metaclass:
class LoggedMeta(type):
def __new__(cls, name, bases, dct):
# Log the creation of every class
print(f"Creating class: {name}")
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=LoggedMeta):
def hello(self):
return "Hello, World!"
The Power of Automation: Class Factory Pattern
One of the most powerful applications of metaclasses is implementing the Class Factory pattern. Imagine you’re building a system where classes need to be created dynamically based on certain rules or configurations.
class ModelMeta(type):
def __new__(cls, name, bases, dct):
# Add automatic field validation
for key, value in dct.items():
if isinstance(value, Field):
dct[f'validate_{key}'] = value.create_validator()
return super().__new__(cls, name, bases, dct)
class Field:
def __init__(self, field_type):
self.field_type = field_type
def create_validator(self):
field_type = self.field_type
def validator(instance, value):
if not isinstance(value, field_type):
raise TypeError(f"Expected {field_type.__name__}")
return validator
class Model(metaclass=ModelMeta):
pass
# Now we can create models with automatic validation
class User(Model):
name = Field(str)
age = Field(int)
Real-World Applications
Metaclasses shine in scenarios where you need to:
- Enforce Coding Standards
class PEP8EnforcerMeta(type):
def __new__(cls, name, bases, dct):
# Enforce PEP 8 naming conventions
if not name[0].isupper():
raise NameError("Class names must start with an uppercase letter")
return super().__new__(cls, name, bases, dct)
- Create Abstract Base Classes
class APIEndpointMeta(type):
def __new__(cls, name, bases, dct):
# Ensure all API endpoints implement required methods
if 'handle_request' not in dct:
raise TypeError(f"{name} must implement handle_request()")
return super().__new__(cls, name, bases, dct)
- Register Classes Automatically
class PluginRegistry(type):
plugins = {}
def __new__(cls, name, bases, dct):
new_cls = super().__new__(cls, name, bases, dct)
# Auto-register plugins
if 'plugin_name' in dct:
cls.plugins[dct['plugin_name']] = new_cls
return new_cls
Best Practices and Pitfalls
While metaclasses are powerful, they should be used judiciously. Here are some guidelines:
- Use Metaclasses Sparingly: They’re best reserved for framework-level code where you need to enforce complex class creation rules.
- Keep Them Simple: A metaclass should have a single, well-defined responsibility.
- Document Thoroughly: Since metaclasses operate at a meta-level, clear documentation is crucial.
- Consider Alternatives: Class decorators or inheritance might be simpler solutions for your use case.
The Power of Introspection
Metaclasses give us unprecedented power to inspect and modify classes at runtime:
class IntrospectiveMeta(type):
def __new__(cls, name, bases, dct):
# Print all methods defined in the class
methods = {name: value for name, value in dct.items()
if callable(value) and not name.startswith('__')}
print(f"Methods in {name}: {list(methods.keys())}")
return super().__new__(cls, name, bases, dct)
Conclusion
Metaclasses represent one of Python’s most sophisticated features, offering unparalleled control over class creation and behavior. While they might seem mysterious at first, understanding metaclasses opens up new possibilities for creating elegant, maintainable, and powerful code architectures.
Remember: with great power comes great responsibility. Use metaclasses when they truly solve your problem in a way that simpler solutions cannot. When used appropriately, they can make your code more elegant, maintainable, and powerful.